drawingTools
The drawingTools()
context manager allows you to create layers using a subset of the DrawBot drawing API. The properties of the layers created with this are not changeable after creation. The creation of these layers can be expensive, so it is not recommended that this be used for complex drawing that needs to be updated frequently.
with container.drawingTools() as bot:
bot.fill(1, 0, 0, 1)
bot.rect(50, 50, 400, 100)
bot.fill(0, 1, 0, 1)
bot.oval(60, 60, 80, 80)
with bot.savedState():
bot.stroke(1, 1, 0, 1)
bot.strokeWidth(10)
bot.lineDash(2, 2)
bot.line((160, 60), (160, 140))
bot.fill(1, 1, 1, 1)
bot.fontSize(22)
bot.textBox(
"Merz " * 15,
(180, 60, 260, 80)
)
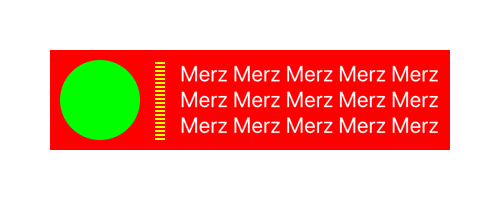
Basic Properties
- MerzDrawingTools.fill(r=None, g=None, b=None, a=1)
Sets the fill color with a red, green, blue and alpha value. Each argument must a value float between 0 and 1.
- MerzDrawingTools.stroke(r=None, g=None, b=None, a=1)
Sets the stroke color with a red, green, blue and alpha value. Each argument must a value float between 0 and 1.
- MerzDrawingTools.strokeWidth(value)
Sets stroke width.
- MerzDrawingTools.lineJoin(join)
Set a line join. Possible values are
"miter"
,"round"
and"bevel"
.
- MerzDrawingTools.lineCap(cap)
Set a line cap. Possible values are
"butt"
,"square"
and"round"
.
- MerzDrawingTools.lineDash(*arg)
Set a line dash with any given amount of lengths. Uneven lengths will have a visible stroke, even lengths will be invisible.
Transformations
These are currently not supported.
- MerzDrawingTools.transform(transform, center=None)
- MerzDrawingTools.translate(x=0, y=0)
- MerzDrawingTools.rotate(angle, center=None)
- MerzDrawingTools.scale(x=1, y=None, center=None)
- MerzDrawingTools.skew(angle1, angle2=0, center=None)
Primitives
- MerzDrawingTools.rect(x, y, w, h)
Draw a rectangle from position x, y with the given width and height.
- MerzDrawingTools.roundedRect(x, y, w, h, radius)
- MerzDrawingTools.oval(x, y, w, h)
Draw an oval from position x, y with the given width and height.
- MerzDrawingTools.line(pt1, pt2)
Draws a line between two given points.
- MerzDrawingTools.newPath()
Create a new path.
- MerzDrawingTools.polygon(xy, *args, **kwargs)
Draws a polygon with n-amount of points. Optionally a
close
argument can be provided to open or close the path. As default a polygon is a closed path.
Paths
- MerzDrawingTools.moveTo(pt)
Move to a point
x
,y
.
- MerzDrawingTools.lineTo(pt)
Line to a point
x
,y
.
- MerzDrawingTools.curveTo(xy1, xy2, xy3)
Curve to a point x3, y3. With given bezier handles x1, y1 and x2, y2.
- MerzDrawingTools.arc(center, radius, startAngle, endAngle, clockwise)
Arc with
center
and a givenradius
, fromstartAngle
toendAngle
, going clockwise ifclockwise
isTrue
and counter clockwise ifclockwise
isFalse
.
- MerzDrawingTools.arcTo(xy1, xy2, radius)
Arc from one point to an other point with a given
radius
.
- MerzDrawingTools.closePath()
Close the path.
- MerzDrawingTools.endPath()
End the path.
- MerzDrawingTools.drawGlyph(glyph)
Draw the given glyph.
- MerzDrawingTools.drawPath(path=None)
Draw the current path, or draw the provided path.
Text
- MerzDrawingTools.text(txt, xy, align='left')
Draw a text at a provided position. Optionally an alignment can be set. Possible align values are:
"left"
,"center"
and"right"
. The default alignment is"left"
. Optionallytxt
can be a Attributed String.
- MerzDrawingTools.textBox(txt, box, align='left')
Draw a text in a provided rectangle. A
box
must be a(x, y, w, h)
. Optionally an alignment can be set. Possiblealign
values are:"left"
,"center"
,"right"
and"justified"
. The default alignment isleft
.
- MerzDrawingTools.font(fontName, fontSize=None)
Set a font with the name of the font. Optionally a
fontSize
can be set directly. The name of the font relates to the font’s postscript name.
- MerzDrawingTools.fontSize(fontSize)
Set the font size in points.
- MerzDrawingTools.lineHeight(value)
Set the line height.
Graphics State
- MerzDrawingTools.savedState()
Save and restore the current graphics state in a
with
statement.