Properties
Layers have properties. These properties tell the layer what it should look like, where it should be positioned and so on. All properties can be set when a layer is created and almost all properties can be changed after the layer has been created.
Properties have a base name, for example “backgroundColor”. Each property has a method for setting its value that is named “set” plus the base name (following camel case conventions), for example “setBackgroundColor”. Likewise, each property has a method for getting its value that is follows the same naming convention with “get” in place of “set”, for example “getBackgroundColor”.
During Creation
Setting a layer property while the layer is being created is accomplished by passing the property name and value as arguments in the construction method:
container.appendBaseSublayer(
backgroundColor=(1, 0, 0, 1)
)
Any number of properties can be passed:
container.appendBaseSublayer(
position=(50, 50),
size=(400, 100),
backgroundColor=(1, 0, 0, 1),
cornerRadius=25
)
It’s even okay to not pass any properties:
container.appendBaseSublayer()
After Creation
Once a layer has been created, you can set the properties as needed:
layer = container.appendBaseSublayer()
layer.setPosition((50, 50))
layer.setSize((400, 100))
layer.setBackgroundColor((1, 0, 0, 1))
layer.setCornerRadius(25)
When a property is set, Merz schedules the change to be displayed immediately. Consequently, if you make many sequential changes, as in the example above, sub-optimal performance may result as display may be called for each property change. When you need to change a batch of properties, you should use the propertyGroup()
context manager:
layer = container.appendBaseSublayer()
with layer.propertyGroup():
layer.setPosition((50, 50))
layer.setSize((400, 100))
layer.setBackgroundColor((1, 0, 0, 1))
layer.setCornerRadius(25)
This will let Merz delay the display scheduling until the last property has been set.
Value Types
Most of the value types that can be set into properties are basic Python types in conventional arrangements. There are a few value types special to Merz:
Color Definitions
Color values are defined as tuples of four float
values between 0 and 1.0. The values correspond with the red, green, blue and alpha values of a rgba color. None
may be used instead of a tuple to indicate that no color should be used.
container.appendBaseSublayer(
backgroundColor=(1, 0, 0, 1),
position=(0, 150),
size=(500, 50)
)
container.appendBaseSublayer(
backgroundColor=(0, 1, 0, 1),
position=(0, 100),
size=(500, 50)
)
container.appendBaseSublayer(
backgroundColor=(0, 0, 1, 1),
position=(0, 50),
size=(500, 50)
)
container.appendBaseSublayer(
backgroundColor=(1, 1, 0, 1),
position=(0, 0),
size=(500, 50)
)
container.appendBaseSublayer(
backgroundColor=(0, 0, 0, 0.5),
position=(100, 0),
size=(100, 200)
)
container.appendBaseSublayer(
backgroundColor=(1, 1, 1, 0.5),
position=(300, 0),
size=(100, 200)
)
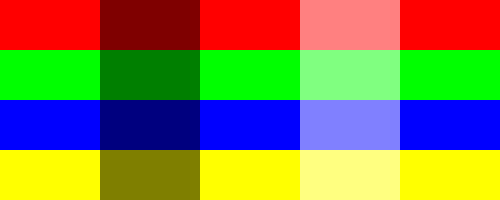
Attributed String
An attributed string is a list of strings and/or dictionaries defining runs of characters with attributes that differ from the attributes defined by the layer level properties. The dictionaries are defined as key value pairs with the following options:
"text"
The text for this run."font"
The font for this run. This has the same options assetFont()
. (optional)"weight"
The weight for this run. This has the same options assetWeight()
. (optional)"pointSize"
The point size for this run. This has the same options assetPointSize()
. (optional)"lineHeight"
The line height for this run This has the same options assetLineHeight()
. (optional)"figureStyle"
The figure style for this run. This has the same options assetFigureStyle()
. (optional)"fillColor"
The fill color for this run. This has the same options assetFillColor()
. (optional)
Not all attributes need to be defined in each dictionary. You only need to define any attribute that you want to be different from the layer level attributes. For example, to only change the weight you would do this:
dict(
text="This is bold text.",
weight="bold"
)
If a string is given instead of a dictionary, the string has the same value as this dictionary:
dict(
text=string
)
In practice, attributed strings are often a mix of dictionaries and strings.
[
"This is regular text. "
dict(
text="This is bold text.",
weight="bold"
),
dict(
text="This is regular, red text. ",
fillColor=(1, 0, 0, 1)
),
"Finally, this is more regular text."
]